Micro Frontends is an architectural style that involves breaking down a web application into smaller, independent, and self-contained units, known as micro frontends. Each micro frontend is responsible for a specific feature or functionality of the application. This approach is inspired by the principles of microservices but applied to the frontend side of the application.
Key characteristics of Micro Frontends:
- Independent Deployment: Each micro frontend can be developed, tested, and deployed independently. This allows teams to work on different parts of the application without interfering with each other.
- Technology Agnostic: Different micro frontends within the same application can be built using different technologies. For example, one micro frontend might be built with React, while another might use Angular or Vue.js. This flexibility allows teams to choose the best technology for their specific needs.
- Isolation: Micro frontends are isolated from each other to minimize dependencies. They communicate through well-defined APIs or protocols. This isolation ensures that a failure in one micro frontend does not affect the entire application.
- Scalability: Teams can scale independently, focusing on specific features or areas of expertise. This allows for faster development cycles and more efficient use of resources.
- Loose Coupling: Micro frontends are loosely coupled, reducing the risk of changes in one part of the application affecting others. This makes it easier to maintain and evolve the application over time.
- Consistent User Experience: Despite being developed independently, micro frontends need to provide a consistent user experience. Shared components, styles, and communication mechanisms are used to achieve this consistency.
- Incremental Upgrades: Upgrading or replacing a specific feature can be done incrementally without affecting the entire application. This is particularly useful for large and complex applications where a complete rewrite might not be feasible.
Micro Frontends are especially relevant in large and complex web applications where multiple teams are involved, and there is a need for agility, scalability, and independent development and deployment.
Micro-frontends don’t follow any particular structure and have no fixed boundaries. Your project will likely evolve as time passes, and you may need to revise your micro-frontend as you go along:
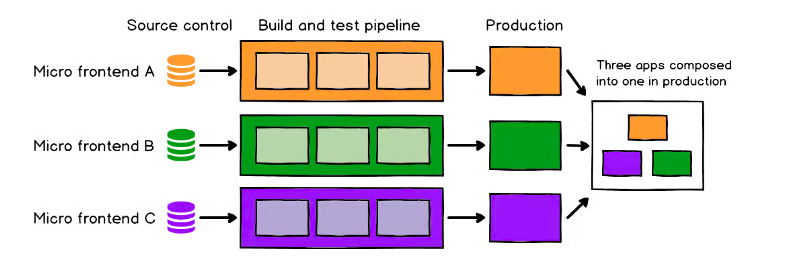
Build Mircro Frontend with React
Building a Micro Frontend application in React involves breaking down a large application into smaller, independently deployable and scalable units. Each micro frontend typically represents a self-contained feature or module. Micro Frontends is an architectural style that involves breaking down a web application into smaller applications.
Below is a simplified guide to help you get started with building a Micro Frontend application using React:
Project Structure:
Organize your project into micro frontend modules. For instance, you can have separate directories for each micro frontend:
/my-app
/microfrontend1
- package.json
- App.js
- ...
/microfrontend2
- package.json
- App.js
- ...
/container-app
- package.json
- App.js
- ...
Setup React Apps:
In each micro frontend directory, set up a React application. You can use create-react-app
or set up your configuration via follow steps here.
npx create-react-app microfrontend1
Shared Components and Styles:
Create shared components and styles that can be reused across micro frontends. This helps maintain a consistent look and feel.
/shared-components
- Button.js
- styles.css
Communication:
Establish communication between micro frontends. You can use techniques like Custom Events, Shared State, or a central state management library like Redux.
Integration:
In the container app (which integrates all micro frontends), use React components to integrate and display micro frontends. Routing can be handled using a library like React Router.
// container-app/src/App.js
import React from 'react';
import Microfrontend1 from './microfrontend1';
import Microfrontend2 from './microfrontend2';
function App() {
return (
<div>
<h1>Container App</h1>
<Microfrontend1 />
<Microfrontend2 />
</div>
);
}
export default App;
Deployment:
Deploy each micro frontend independently. You can use technologies like Docker, Kubernetes, or serverless architecture depending on your deployment environment.
A notable benefit of employing the micro-frontend architecture is the ability to disassemble a unified structure into distinct components, allowing for independent deployments. Vercel facilitates the support of separate repositories containing diverse frontends, irrespective of the language or framework, enabling their cohesive deployment. Alternatively, deployment platforms such as Netlify can be utilized. Following deployment of the micro-frontend, it can be utilized exclusively as an independent frontend module.
CI/CD:
Set up Continuous Integration and Continuous Deployment pipelines for each micro frontend and the container app.
Monitoring and Logging:
Implement logging and monitoring solutions to keep track of the performance and health of your micro frontend application.
Remember that this is a simplified guide, and depending on your specific requirements, you might need to adapt or extend these steps. Also, consider using frameworks and tools designed for micro frontend architectures, such as single-spa or Module Federation in Webpack 5.